Stack vs Heap Allocation in C: Pros and Cons
In this blog post, we will explore the differences between stack and heap allocation, their advantages, disadvantages, and when it's appropriate to use each one.
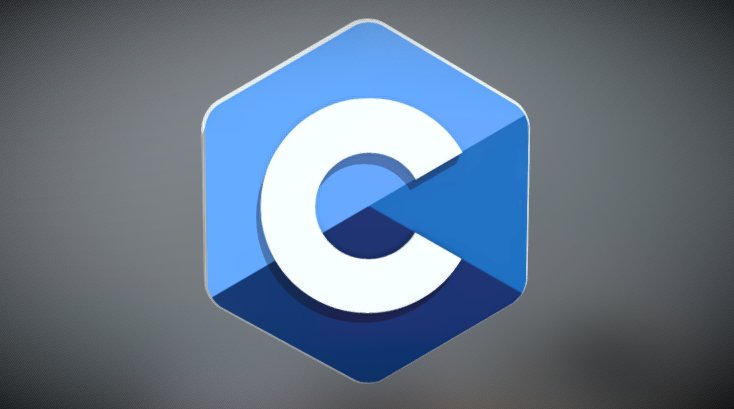
Introduction:
Memory management plays a critical role in software development, especially in low-level programming languages like C. There are two basic ways for allocating memory in C: stack allocation and heap allocation.
Understanding the pros and cons of stack and heap allocation will help you write high-performance code, be able to run your code in extremely constrained environments like embedded devices, and avoid costly bugs and mistakes.
Stack Allocation
The stack is a small section of memory that is managed by the operating system. For example, it is usually about 1MB in Windows systems, and 8MB in Linux systems.
When you declare a scalar variable inside a function or a block, it is typically allocated on the stack. With arrays, on the other hand, you have to be explicit about your decision.
Stack allocation refers to the automatic allocation and deallocation of memory on the stack. The stack is a region of memory managed by the compiler, and
Importantly, stack allocation and deallocation happen automatically as you enter and exit the scope of the variable, and this means that the stack is designed to be used with small, temporary arrays.
In order to allocate an array on the stack in C, the notation is pretty straightforward:
double numbers[ARRAY_SIZE];
where ARRAY_SIZE
could be a constant known at compile time, or, by relying on a feature of the C99 standard known as variable-length arrays (VLA), could also be a run-time variable.
Advantages:
- Speed: Stack allocation is generally faster than heap allocation since it involves simple pointer manipulation and does not require complex memory management.
- Deterministic behavior: Memory allocated on the stack is automatically deallocated when it goes out of scope, ensuring deterministic memory management.
- Memory locality: Stack memory is contiguous, resulting in better memory locality and cache performance.
Disadvantages:
- Limited size (and potential stack overflow errors). The stack has a limited size, with a bound that depends on the operating systems of usually a few megabytes. Allocating more memory on the stack can lead to stack overflow errors.
- Static allocation: Unless you are relying on C99 VLAs, the size of stack-allocated variables must be known at compile-time, making it challenging to allocate dynamic memory or large data structures. If you are relying on VLAs, the first isuue is potentially more problematic, as the chances of exceeding the stack size increases.
Let’s illustrate the main disadvantage of stack allocation by producing a program that will lead to a stack overflow:
#include <stdio.h>
#include <stdlib.h>
#define ARRAY_SIZE 800
int main() {
// Define a stack-allocated arrays
double numbers[ARRAY_SIZE];
// Initialize array elements
for (int i = 0; i < ARRAY_SIZE; i++) {
numbers[i] = (double) 1/(i + 1);
}
// Print array elements
for (int i = 0; i < ARRAY_SIZE; i++) {
printf("%f ", numbers[i]);
}
return 0;
}
Depending on your operating system, having ARRAY_SIZE
defined as 8 million as above could lead to a segmentation fault. If you don’t get a segmentation fault when running this program on your system, try with a larger number.
Heap Allocation:
Heap allocation involves manually managing memory on the heap, a larger region of memory available to the program. Memory allocation on the heap is typically done using functions like malloc()
and calloc()
(to force contiguous allocation) and must be explicitly deallocated using free()
when it is no longer needed.
For example, we could allocate our numbers
variable on the heap in C with the following line:
double* numbers = (double*)malloc(ARRAY_SIZE * sizeof(double));
When not required anymore, the memory has to bee manually freed using free
free(numbers)
A very similar result to malloc
and free
can be obtained in C++ using new
and delete
, but this is not part of the C standard.
If you want, try to modify the above program to use the heap and avoid the stack overflow.
Advantages of heap allocation:
- Dynamic memory: The heap allows you to allocate memory dynamically at runtime, enabling you to handle situations where the memory requirements are unknown or change during program execution.
- Flexible memory management: Heap allocation provides flexibility in managing memory. You can allocate and deallocate memory as needed, allowing for the creation of resizable data structures.
- Large memory space: The heap provides a larger memory space compared to the stack, allowing for the allocation of large data structures.
Disadvantages:
- Slower performance: Heap allocation involves more overhead due to memory management operations, such as searching for available memory blocks and bookkeeping.
- Manual memory management: Heap-allocated memory must be explicitly deallocated to prevent memory leaks. Improper management can lead to memory leaks, fragmentation, or dangling pointers.
- Fragmentation: Frequent allocation and deallocation of memory on the heap can result in memory fragmentation, where the heap becomes divided into small, non-contiguous blocks, reducing overall memory efficiency.
For a performance comparison of heap vs stack, and also C++ vectors, check out my post on array performance in C++
Choosing the Right Approach
When deciding between stack allocation and heap allocation, consider the following guidelines:
Use stack allocation when:
- You need fast and deterministic memory management.
- Memory requirements are small and can be bounded at compile-time.
- Lifetime of the variable is well-defined within the scope of a function or a block.
- When you don’t have a choice, like in embedded devices.
Use heap allocation when:
- Memory requirements exceed the limitations of the stack.
- Memory requirements are unknown or vary during runtime.
- You need dynamic data structures that can grow or shrink.
Conclusion
Both stack allocation and heap allocation serve specific purposes in C programming.
Stack allocation offers speed, determinism, and efficient memory usage for small, well-defined variables.
On the other hand, heap allocation provides the ability to handle larger data structures, and also more flexibility, and dynamic memory management.
Further Reading: Advanced Memory Allocation Strategies
So far we have only scratched the surface of what’s possible in C in terms of memory management. For more advanced techniques like implementing custom allocators, an excellent reference is this series of posts by Bill Ginger.