3 Python Packages for the Yahoo Finance (unofficial) API
Get started with stock market data analysis in Python using yfinance, yahooquery, or yahoo_fin.
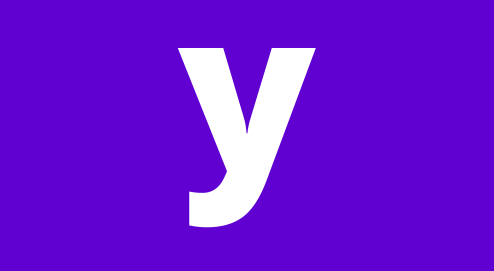
Financial data is essential for developers, analysts, and traders, and Yahoo Finance is a great source of free stock market data. In this post, we will introduce three popular Python libraries that allow you to interact with Yahoo Finance: yfinance
, yahooquery
, and yahoo_fin
.
Bear in mind that all three packages are unofficial and rely on scraping the Yahoo Finance website. If anything changes in Yahoo, including policy changes and restrictions on available data, these three tools will stop working until fixes are made by the community (if possible).
So, while these tools are good for simple use-cases or quick explorations, other options are probably needed for professional use. For a solid and professional API with a very generous free-tier, I recommend checking out AlphaVantage.
Let’s now quickly review the scraper packages.
1. yfinance
yfinance
on GitHub | Documentation
yfinance
is the most popular Python package for retrieving Yahoo Finance data. It simplifies the process of downloading historical stock prices, dividends, stock splits, and other data directly from Yahoo Finance.
Key Features:
- Historical Market Data: Easily download daily prices, adjusted close prices, dividends, and stock splits.
- Financial Data: Fetch financial statements such as balance sheets and cash flow statements.
- Simple Interface: Its simplicity makes it ideal for beginners who need quick access to stock data.
- Built-in Pandas Integration:
yfinance
directly outputs data in a Pandas DataFrame, allowing for easy data manipulation and analysis.
Example Usage:
First install it using pip (or conda)
pip install yfinance
Let’s now get historical prices
import yfinance as yf
# Download historical data for Apple
ticker = yf.Ticker("AAPL")
hist_data = ticker.history(period="5y")
print(hist_data.head())
yfinance is a great tool if you’re looking for ease of use, especially for simple historical stock price retrieval.
2. yahooquery
yahooquery
on GitHub | Documentation
yahooquery
is another Python package for Yahoo Finance, but it’s designed to be more performant and feature-rich than yfinance
. One of its key advantages is asynchronous support, making it faster when you need to handle large numbers of tickers.
Key Features:
- Fast: Data is retrieved through API endpoints instead of web scraping. Additionally, asynchronous requests can be utilized with simple configuration
- Simple: Data for multiple symbols can be retrieved with simple one-liners
- User-friendly: Pandas Dataframes are utilized where appropriate
- Premium: Yahoo Finance premium subscribers are able to retrieve data available through their subscription
Example Usage:
Let’s install it using pip (or conda)
pip install yahooquery
from yahooquery import Ticker
# Get data for Apple
ticker = Ticker("AAPL")
hist_data = ticker.history(period="5y")
print(hist_data.head())
If you’re dealing with a large number of tickers or need access to premium-subscribers data, yahooquery is an excellent choice.
3. yahoo_fin
yahoo_fin
on GitHub | Documentation
yahoo_fin
is another package for scraping Yahoo Finance data
It is the least popular of the three, the least frequently updated so it’s more suceptible of bugs, and doesn’t offer any unique features that I’m aware of, so it is only included in this list for completeness.