For Loops
Organizing our code with simple loops, nested for loops, and parallel for loops.
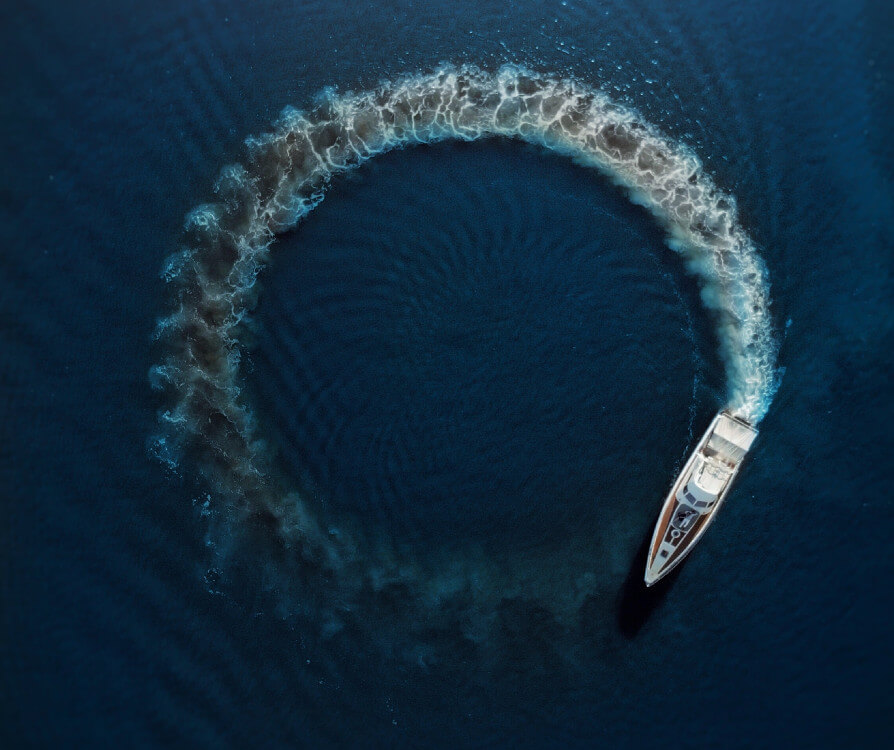
Simple For Loop
The most basic way to structure computations is by using a for loop. In Julia, the basic syntax of a for loop is
for iterator in range
execute_statements(iterator)
end
As an example, let’s evaluate the sum of the first 100,000 terms of the quadratic series.
x=0
for k in 1:100000
x = x + (1/k)^2
end
We get the following output
1.6449240667982423
Note on pre 1.5 Julia versions. This code, when executed from a REPL, doesn’t work for Julia versions between 1.0 and 1.5, as some changes were introduced at those points in relation to so-called variable scoping (see documentation on Soft Scoping). The presented code does work for Julia 1.6, which, at the time of writing this, is the long-term-supported (LTS) version of Julia. Previous versions of Julia are now considered obsolete. If you need to upgrade your version of Julia, head over to the download page. To find out which version of Julia you are using simply type VERSION
in the REPL.
Nested For Loops
It is very common to have nested for loops, and we would normally code them using something like:
for i in 1:3
for j in 1:3
print("i=", i, " j=", j, "\n")
end
end
This can get hard to read when we have more than two nested loops. A nice syntax we can rely on in this case is the following:
for i in 1:3, j in 1:3
print("i=", i, " j=", j, "\n")
end
Additionally, we can resort to the even nicer:
for i ∈ 1:3, j ∈ 1:3
print("i=", i, " j=", j, "\n")
end
Break and Continue Statement
The use of break and continue statements is somewhat contrived, and could be considered bad programming practice by some people. However, I believe break and continue statements are harmless when used carefully, and could even be preferable in some cases.
Break Statement Example
The Break statement is used to exit a for-loop before it has completed all its iterations, usually after checking that a certain condition is met.
For example, in a numerical algorithm, we could be iterating until a certain number of iterations is met, but we could interrupt the computations if some convergence criteria is met.
Let’s compute the sum of a series to include a break statement as follows:
x=0
for k in 1:100000
term = (1/k)^2
x = x + term
if (abs(term) < 1e-10) break end
end
While its possible to rewrite the above code with the sole purpose of avoiding the use of the break statement, we might need to write something like this:
x=0
iter = 0
while ( iter == 0 || abs(term) < 1e-10) && (iter < 100000)
term = (1/k)^2
x = x + term
iter = iter + 1
end
Continue Statement Example
The Continue statement is used to exit the present iteration of a for-loop, and to continue with the next iteration immediately.
A reasonable use-case for the continue statement is as some sort of precondition-test for a bad argument passed to a loop.
For example, let’s say we have a function that sums the inverses of a set of random numbers. Logically, we would like to avoid summing 1/0
, as that would result in Inf
and could lead to errors.
One way to avoid this undesired input is to use a continue statement.
numbers = randn(100)
sum = 0
for k in numbers
if (k==0) continue end
sum = sum + 1/k
end
One alternative, without the continue statement, could be as follows:
numbers = randn(100)
sum = 0
for k in numbers
if (k != 0)
sum = sum + 1/k
end
end
In some cases, when we have to check for multiple conditions, the presence of multiple nested-if statements could turn out quite annoying, and the continue statement could be preferred.